Instructor
Java Programming Course | Learn Java for Development
Curriculum
Enroll in our Java Programming course to master object-oriented programming, data structures, algorithms, and frameworks. Learn to build scalable applications with Java for web and mobile development. Join now!
Ratings
( 4.1 Ratings )
Live Online Classes starting on 01 January, 1970
SpireTec Unique Features
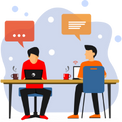
1-On-1 Training
Benefit from our 1-On-1 Training for personalized, focused, and effective learning experiences.

Customized Training
Experience our Customized Training service tailored to meet your specific learning needs and goals
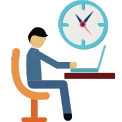
4 - Hours / Weekend Session
Join our Class featuring 4 - Hours / Weekend Session for in-depth learning and expert training.

Free Demo Class
Join our Free Demo Class to experience top-notch training and expert guidance first hand!
Purchase This Course
Request More Information
CERTIFICATE
Get Ahead With
SpireTec Solutions
Training Certificate
Earn your Certificate
Our course is exhaustive and this certificate is proof that you have taken a big leap in mastering the domain.
Differentiate yourself with Masters Certificate
Our course is exhaustive and this certificate is proof that you have taken a big leap in mastering the domain.
Share your achievement
Our course is exhaustive and this certificate is proof that you have taken a big leap in mastering the domain.
Need Customized Curriculum?
Our course is exhaustive and this certificate is proof that you have taken a big leap in mastering the domain.
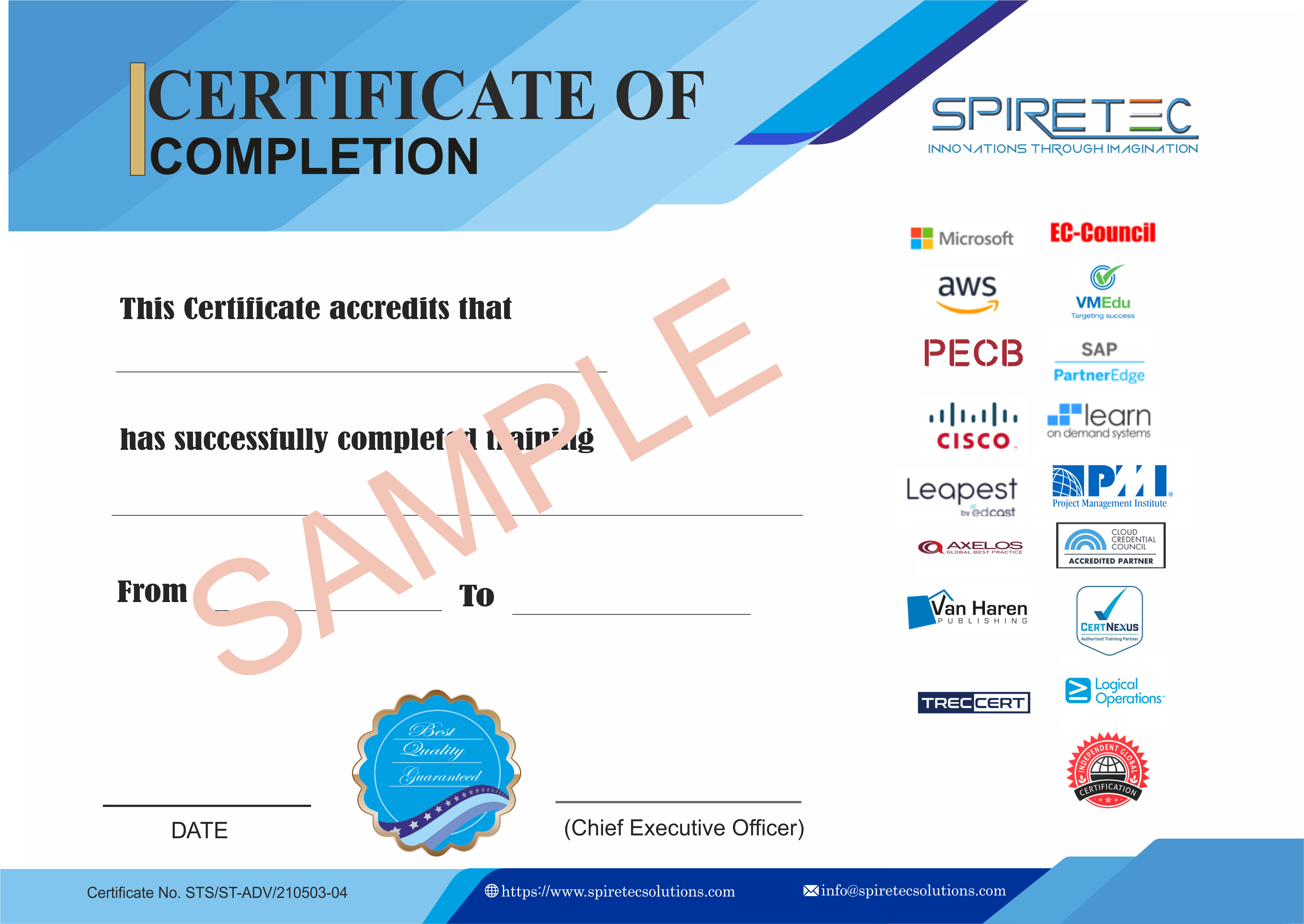